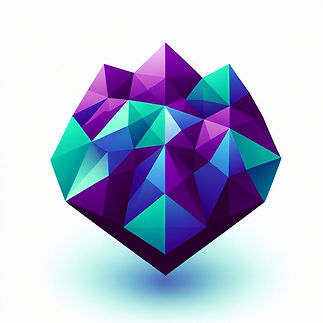
VS Code and basics of C
In this blog, we focus on mastering the basics of C programming while using VS Code, a widely-used, efficient code editor. You'll learn how to write clean, effective C code. We’ll guide you through setting up VS Code for C development, offering tips for writing, compiling, and debugging programs. Whether you're just starting or looking to improve your skills, this blog will help you build a strong foundation in C programming using one of the best code editors available.
How to set up VS Code for C
Setting up the development environment for C involves choosing an Integrated Development Environment (IDE) or using a simple text editor along with a compiler. Here are steps for setting up VS Code.
Steps to be taken to set up it perfectly
-
Download VS Code [code editor specified to your system] (VS Code setup)
-
Install VS Code (Double on the setup to run and then follow the installation wizard steps).
-
Open VS Code and click on the extensions icon or press shortcut (Ctrl+shift+x) and search for "c" and then install [C/C++ IntelliSense, debugging, and code browsing].by. Microsoft.
-
Search in the browser and download Min GW C compiler or follow the link Compiler
-
Follow the installation wizard steps, Click to install Min GW ⇾run ⇾wait ⇾continue ⇾check all the boxes or only c++ compiler one(g++) ⇾look at left of the same window and click installation tab ⇾click apply changes ⇾wait for completion ⇾close
-
go to c:\disk and Min GW directory ⇾bin ⇾click inside the address bar and copy address like (C:\MinGW\bin)
-
Press window logo key and search [env](edit system environment variables) ⇾click on environment variables ⇾look for path inside system variables[user variable] ⇾select path and click edit then click ⇾new ⇾past the copied path (C:\MinGW\bin) ⇾ok ⇾ok ⇾ok
-
Run VS Code and Create a basic c program and save it with .c extension.
-
To run your code run a new terminal in your VS Code (top menu bar) and write ⇾gcc yourprogramname.c then Press Enter [for compilation] OR Install .run extension and then press it's run icon (like a video play icon) from the top right of your VS Code.
-
After compilation, Enter (a)[in the same terminal] and then press tab to auto complete the default .exe file name [to run your code].
In case you got errors, you can always watch tutorials!

Programmer
A machine that turns coffee into code.
Basic Concepts of C programming
Here we will discuss and understand the basic concepts of c programming, covering syntax, structure of C program, data types, variables and operators. In depth detail, just scroll down and go through each.
Syntax and Structure
Before diving into coding, it's essential to understand the basic syntax and structure of a C program. Think of syntax as the grammar and structure as the framework that holds everything together.
Hello World! Program in C
The "Hello, World!" program is the most straightforward example to illustrate the basic syntax of a C program. Here’s what it looks like:
Breaking It Down
-
#include <stdio.h>: This line includes the Standard Input Output library in your program, allowing you to use functions like printf. The #include is a preprocessor directive, meaning it runs before the actual compilation process begins.
-
int main(): Every C program must have a main function. This is where the execution starts. The int before main signifies that this function will return an integer value (0 in this case).
-
{ } (Curly Braces): These define the start and end of the main function. All the code that belongs to main goes inside these braces.
-
printf("Hello, World!\n");: This line prints the text "Hello, World!" to the console. The \n is a newline character, meaning the cursor will move to the next line after printing.
-
return 0;: This statement exits the main function and returns the value 0 to the operating system, indicating that the program ran successfully.
Data Types and Variables
Data types and variables are fundamental building blocks in any programming language, including C. They determine the kind of data you can store and manipulate.
Common Data Types in C
-
int (Integer): Used to store whole numbers, both positive and negative.
-
For example:
int age = 25;
-
float (Floating-point): Used for numbers with decimals.
-
For example:
float price = 19.99;
-
char (Character): Used to store a single character.
-
For example:
char grade = 'A';
-
double (Double-precision floating-point): Like float but with more precision.
-
For example:
double pi = 3.1415926535;
-
void: Represents the absence of a data type, often used for functions that do not return a value.
Variables:
Variables are containers for storing data. When you declare a variable, you specify its type and give it a name. For example:
Understanding Variable Declaration and Initialization:
-
Declaration: Stating the type and name of the variable.
-
E.g., int age;
-
-
Initialization: Assigning a value to the variable.
-
E.g., age = 25;
-
You can also declare and initialize in one step: int age = 25;
Operators
Operators are symbols that tell the compiler to perform specific mathematical, relational, or logical operations.
Types of Operators:
-
Arithmetic Operators: Used for basic mathematical operations.
-
+ (Addition): Adds two values. E.g., int sum = 5 + 3; // sum is 8
-
- (Subtraction): Subtracts one value from another. E.g., int difference = 5 - 3; // difference is 2
-
* (Multiplication): Multiplies two values. E.g., int product = 5 * 3; // product is 15
-
/ (Division): Divides one value by another. E.g., int quotient = 6 / 3; // quotient is 2
-
% (Modulus): Returns the remainder of a division. E.g., int remainder = 5 % 2; // remainder is 1
-
-
Relational Operators: Compare two values and return true or false.
-
== (Equal to): Checks if two values are equal. E.g., a == b
-
!= (Not equal to): Checks if two values are not equal. E.g., a != b
-
> (Greater than): Checks if one value is greater than the other. E.g., a > b
-
< (Less than): Checks if one value is less than the other. E.g., a < b
-
>= (Greater than or equal to): Checks if one value is greater than or equal to the other. E.g., a >= b
-
<= (Less than or equal to): Checks if one value is less than or equal to the other. E.g., a <= b
-
-
Logical Operators: Used to perform logical operations, often with relational operators.
-
&& (Logical AND): Returns true if both conditions are true. E.g., (a > b) && (c > d)
-
|| (Logical OR): Returns true if at least one condition is true. E.g., (a > b) || (c > d)
-
! (Logical NOT): Reverses the logical state of its operand. E.g., !(a > b) is true if a is not greater than b.
-
-
Bitwise Operators: Used to perform bit-level operations.
-
& (AND): Performs a bitwise AND operation.
-
| (OR): Performs a bitwise OR operation.
-
^ (XOR): Performs a bitwise XOR operation.
-
~ (NOT): Performs a bitwise NOT operation.
-
<< (Left shift): Shifts bits to the left.
-
>> (Right shift): Shifts bits to the right.
-
Example: Combining Operators
You can combine different operators to perform complex operations:
In this example, a + b is calculated first, giving 15, and then it’s multiplied by 2 to give 30.
This detailed overview of syntax and structure, data types and variables, and operators should provide beginners with a solid foundation in C programming. Each concept builds on the previous one, creating a comprehensive understanding of how C works. With these basics mastered, readers will be well-equipped to tackle more advanced topics in their programming journey.
Contact
I'm always looking for new and exciting opportunities. Let's connect.